In this post, we’ll explore the design and implementation of mobile app Web APIs, covering everything from RESTful principles to security considerations. We’ll also dive into practical tips for integrating APIs into iOS and Android apps, helping you create more efficient and feature-rich mobile applications.
What Are Web APIs for Mobile Apps?
The Foundation of Modern Mobile Development
Web APIs form the cornerstone of contemporary mobile app development. These interfaces act as invisible bridges, connecting apps to a vast array of online services and data. They enable developers to create powerful, feature-rich applications without starting from scratch.
Web APIs: Defining the Digital Dialogue
Web APIs (Application Programming Interfaces) are sets of protocols and tools that allow different software applications to communicate over the internet. For mobile apps, they serve as gateways to external data and services, significantly expanding an app’s capabilities.
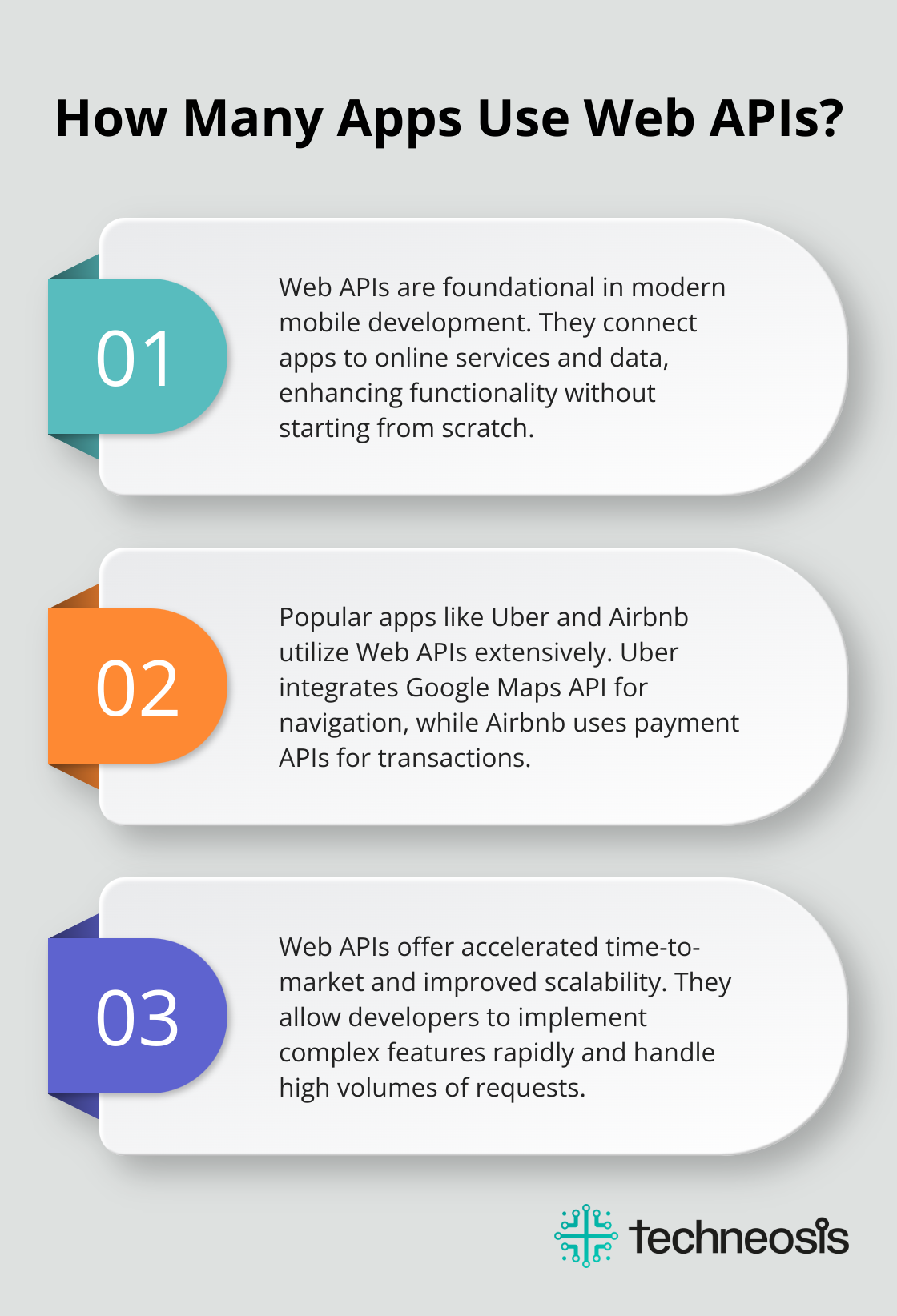
Popular apps like Uber and Airbnb exemplify the power of Web APIs. Uber integrates Google Maps API for navigation, while Airbnb uses payment APIs for transactions. This approach allows developers to focus on creating unique user experiences rather than reinventing existing functionalities.
The Strategic Advantage of Web APIs
The adoption of Web APIs in mobile app development offers substantial benefits.
One primary advantage is the accelerated time-to-market. Leveraging existing APIs allows developers to implement complex features rapidly, reducing development costs and enabling quick prototyping and iteration.
Scalability presents another key benefit. Web APIs are designed to handle high volumes of requests, facilitating easier growth without concerns about backend infrastructure. This aspect proves particularly valuable for startups aiming for rapid expansion.
Web APIs vs. Native APIs: A Comparative Analysis
While both Web APIs and Native APIs facilitate software communication, they differ in significant ways that impact mobile app development.
Web APIs offer platform independence and can be accessed via the internet, making them ideal for cross-platform development. They allow for easier updates and maintenance, as changes can be implemented server-side without requiring app updates.
Native APIs, conversely, are specific to the device’s operating system and provide deeper integration with device features. They often deliver superior performance for tasks requiring intensive device-level access (e.g., camera controls or sensor data).
A hybrid approach often yields the best results. This strategy combines the flexibility of Web APIs for data-driven features and third-party integrations with the performance of Native APIs for on-device functionalities.
The Evolution of Web APIs in Mobile Development
As mobile apps grow in sophistication, Web APIs continue to play an increasingly vital role. A shift towards more specialized APIs, such as those for AI and machine learning, is emerging, paving the way for even more advanced mobile app features.
Furthermore, the rise of IoT (Internet of Things) is creating new opportunities for mobile apps to interact with smart devices through Web APIs. This trend promises to transform how we engage with our environment via mobile devices.
As we move forward, the next chapter will explore the intricacies of designing effective Web APIs for mobile apps, focusing on RESTful principles, performance optimization, and security considerations.
How to Design Mobile-Friendly Web APIs
Embracing RESTful Principles
RESTful design principles promote simplicity, modularity, and independence between client and server components. When you design your API, create clear, intuitive endpoints that map directly to resources. For example, use /users/{id} with appropriate HTTP methods (GET, POST, PUT, DELETE) instead of /getUser.
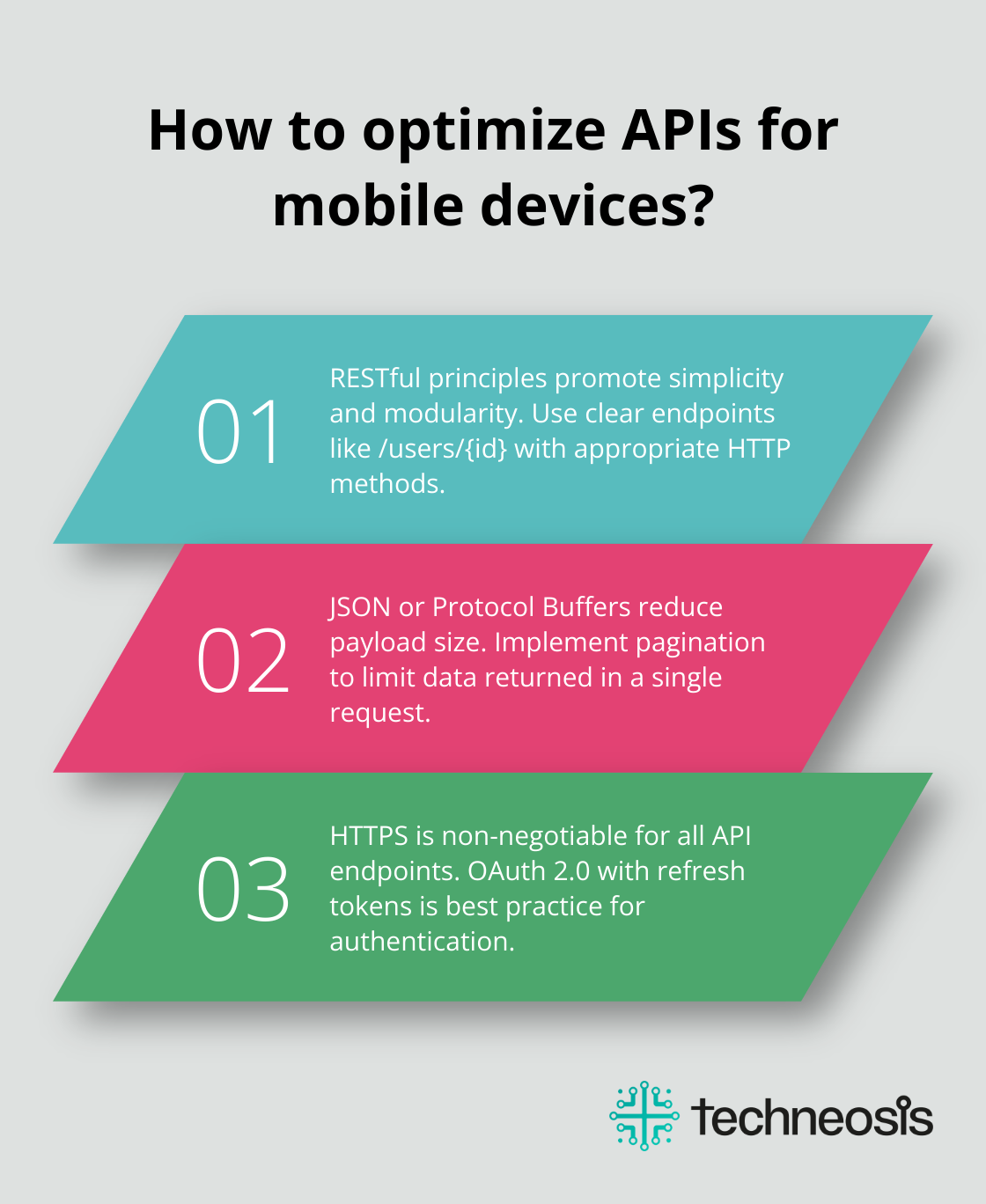
Implement proper HTTP status codes to communicate effectively with mobile clients. This practice helps in error handling and improves the overall reliability of your app.
Optimizing for Mobile Performance
Mobile devices often operate in challenging network conditions. To address this, we recommend you implement efficient data serialization formats like JSON or Protocol Buffers. These formats significantly reduce payload size, which leads to faster data transfer and lower battery consumption.
Pagination is another critical feature for mobile APIs. Limit the amount of data returned in a single request to improve load times and reduce memory usage on mobile devices. Cursor-based pagination works by returning a pointer to a specific item in the dataset, which has proven effective for mobile clients.
Implement a caching strategy to minimize unnecessary network requests. ETags and conditional requests can help mobile apps determine if local data is still valid, which reduces data usage and improves app responsiveness.
Prioritizing Security in Mobile Environments
Security takes top priority in mobile API design. Start by implementing HTTPS for all API endpoints – this is non-negotiable in today’s threat landscape. OWASP lists insecure API endpoints among the top security risks for mobile applications.
For authentication, OAuth 2.0 with refresh tokens is widely considered best practice. The OAuth2 standard defines four grant types (or flows) to request and get an access token, with Spotify implementing several of these in their API.
Rate limiting is another important security measure. It protects your API from abuse and ensures fair usage across all clients. Implement intelligent rate limiting that considers the unique patterns of mobile usage (such as burst requests during app startup).
Don’t overlook the importance of input validation. Mobile environments can be unpredictable, and thorough server-side validation is essential to prevent injection attacks and other security vulnerabilities.
Future-Proofing Your API Design
As mobile technology evolves, so should your API design. Stay informed about emerging trends and technologies that could impact mobile API development. For instance, the rise of 5G networks may influence how we optimize data transfer for mobile devices.
Consider implementing versioning in your API from the start. This allows you to make changes and improvements without breaking existing client integrations. Companies like Stripe have successfully used this approach to maintain backwards compatibility while continuously improving their APIs.
The next chapter will explore the practical aspects of implementing these design principles in real-world mobile app development scenarios. We’ll discuss choosing the right API architecture, best practices for integration in iOS and Android apps, and effective testing strategies.
Implementing Web APIs in Mobile Apps
Selecting the Right API Architecture
The choice of API architecture significantly impacts your app’s performance and user experience. REST (Representational State Transfer) remains a popular choice due to its simplicity and scalability. It excels in apps that require straightforward CRUD (Create, Read, Update, Delete) operations.
GraphQL has gained significant traction, especially for apps with complex data requirements. It allows clients to request specific data, which reduces over-fetching and under-fetching issues common in REST APIs. GraphQL’s support for mobile and web applications is evident in its ability to reduce data transfer, enhance performance, and cater to diverse needs. It’s power also opens it up to many security vulnerabilities though, so it needs to be used with caution. We do not recommend GraphQL for the majority of apps.
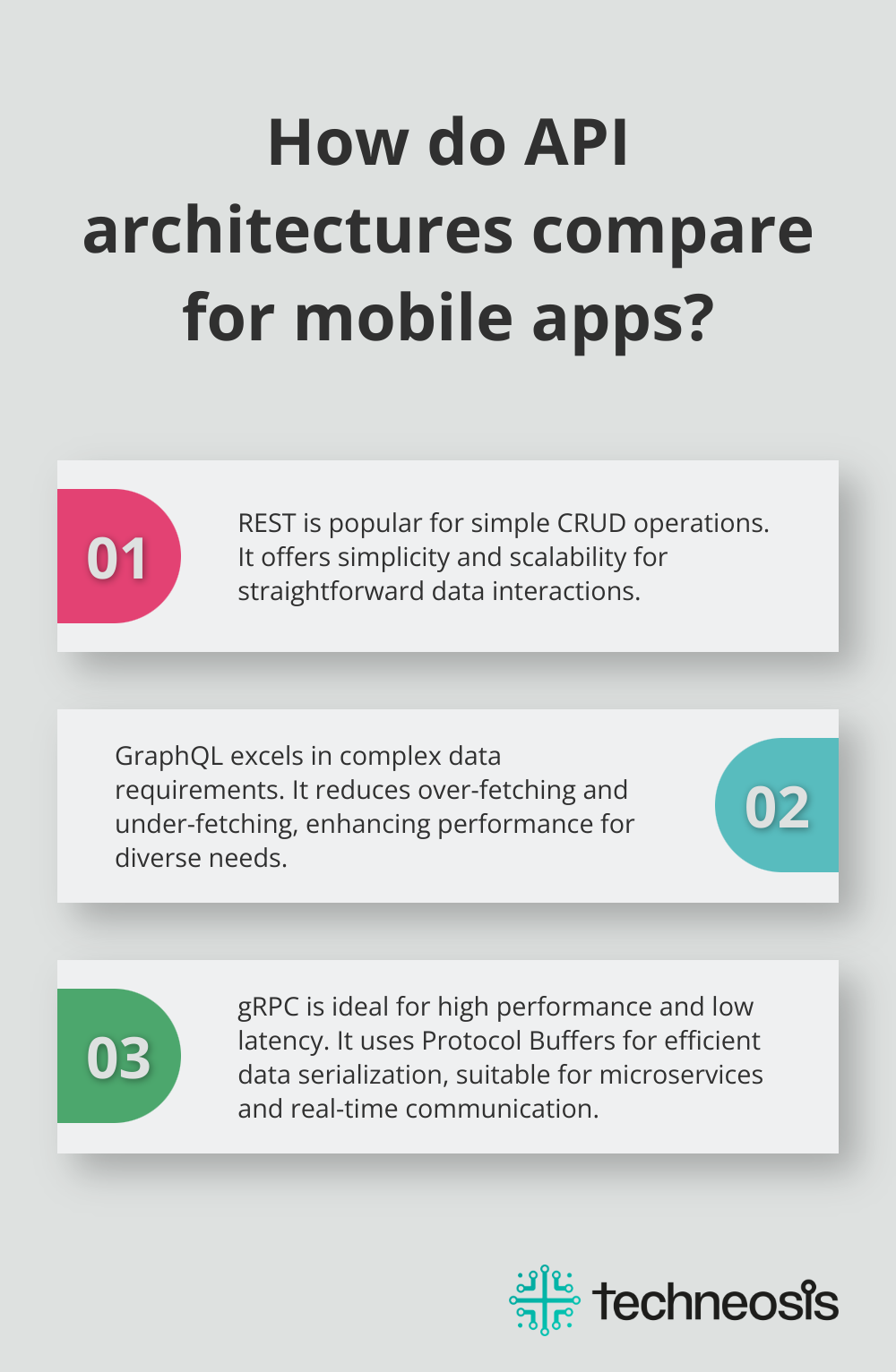
For apps that demand high performance and low latency, gRPC (gRPC Remote Procedure Call) is a strong contender. It uses Protocol Buffers for efficient data serialization, making it ideal for microservices architectures and real-time communication apps.
Integration Best Practices for iOS and Android
When integrating APIs into iOS apps, use Swift’s powerful networking libraries like URLSession or third-party frameworks such as Alamofire. These tools simplify API calls and error handling. For Android, Retrofit has become the standard for API integration, offering type-safe HTTP clients and easy JSON parsing.
Implement proper error handling and retry mechanisms to ensure your app manages network failures or API downtime gracefully. Hystrix is a library designed to control the interactions between distributed services, providing greater tolerance of latency and failure.
Optimize for offline scenarios by implementing local caching strategies. This approach improves app performance and enhances user experience in low-connectivity situations. Square’s Retrofit Cache Control interceptor offers a great starting point for implementing efficient caching in Android apps.
Effective Testing and Debugging Strategies
Thorough testing ensures reliable API implementations. Use tools like Postman or Insomnia to test API endpoints before integration. These tools allow you to simulate various scenarios and validate responses.
For iOS, XCTest provides a robust framework for unit testing API calls. On Android, Espresso and Mockito are excellent choices for UI and unit testing (respectively). Implement continuous integration (CI) pipelines to automate these tests, ensuring API compatibility with each code change.
Debugging API issues can challenge developers, especially in production environments. Implement comprehensive logging and monitoring solutions. Tools like New Relic or Datadog can provide valuable insights into API performance and help identify bottlenecks.
Consider implementing feature flags for gradual API rollouts. This approach allows you to test new API versions with a subset of users before full deployment, which minimizes the impact of potential issues.
Stay vigilant about API versioning. As your app evolves, you’ll likely need to update your API. Implement a clear versioning strategy to maintain backward compatibility while introducing new features. Stripe’s API versioning approach serves as an excellent model, allowing developers to specify the desired API version with each request.
To enhance user trust and protect your app effectively, it’s crucial to implement robust security measures throughout the API integration process.
Final Thoughts
Web APIs for mobile apps have revolutionized modern app development. They enhance functionality, improve user experiences, and provide developers with powerful tools to create robust applications. The landscape of mobile API development evolves rapidly, with shifts towards specialized APIs in areas like artificial intelligence and machine learning.
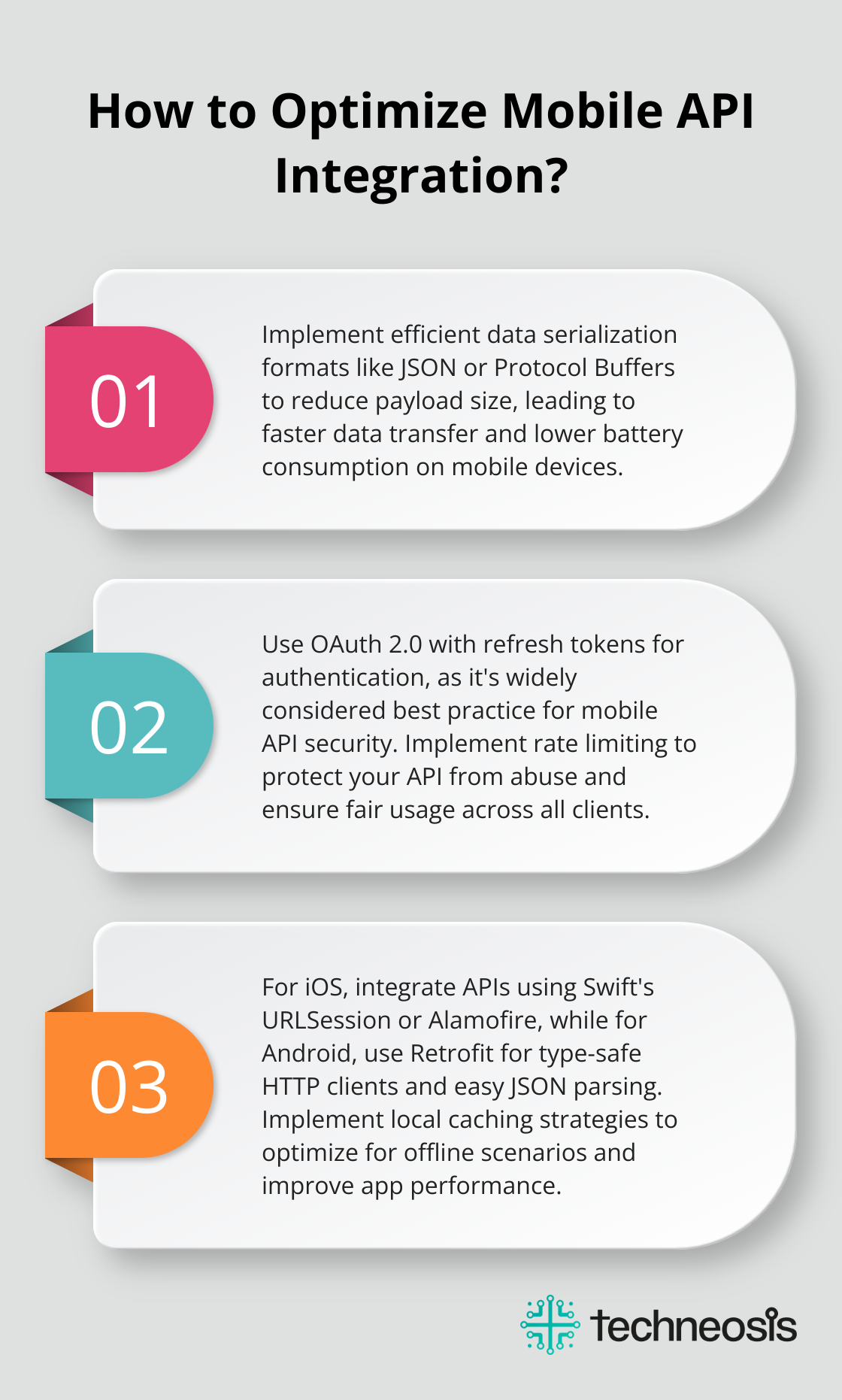
Developers must adapt to these changes and stay informed about emerging trends and security best practices. This continuous learning ensures the creation of cutting-edge mobile applications that meet current needs and prepare for future technological advancements. The importance of mobile app Web APIs will only grow as technology progresses.
We at Techneosis understand the complexities of modern app development. Our team helps businesses navigate these challenges and leverage the power of Web APIs in their mobile applications. As the field advances, embracing best practices in API design and implementation will remain key to creating successful mobile apps.