In this post, we’ll explore how to implement React Native app state change listeners and leverage them for practical use cases. We’ll cover everything from basic setup to advanced techniques for optimizing your app’s performance.
Understanding App State in React Native
What is App State?
App state in React Native represents the current condition of your application at any given moment. It encompasses not only the data your app holds but also how it behaves in different scenarios. Proper app state management can significantly impact an application’s user experience.
The Three Key App States
React Native primarily deals with three app states:
- Active: Your app runs in the foreground and receives user input.
- Background: Your app runs behind other apps or when the device is locked.
- Inactive: A brief transitional phase between active and background states.
Importance of App State Management
Effective app state management is essential for several reasons:
- It enables your app to respond appropriately to system events (e.g., incoming calls or notifications).
- It optimizes battery life by managing resources efficiently when your app is not in active use.
- It ensures data integrity by syncing or saving important information when the app moves to the background.
The AppState API
React Native’s AppState API provides powerful tools for monitoring and responding to state changes. You can use AppState.currentState to check the current state of your app, or AppState.addEventListener to set up listeners for state changes. AppState can tell you if the app is in the foreground or background, and notify you when the state changes.
Practical Applications
App state management has numerous practical applications. You can use it to:
- Pause video playback when the app goes to the background
- Trigger a data sync when the app becomes active again
- Manage push notifications (ensuring they’re handled appropriately regardless of whether your app is in the foreground or background)
Performance Considerations
While app state management is important, you must implement it efficiently. Overusing listeners or performing heavy operations during state changes can lead to performance issues. We recommend using debounce techniques and ensuring that your state change handlers are as lightweight as possible.
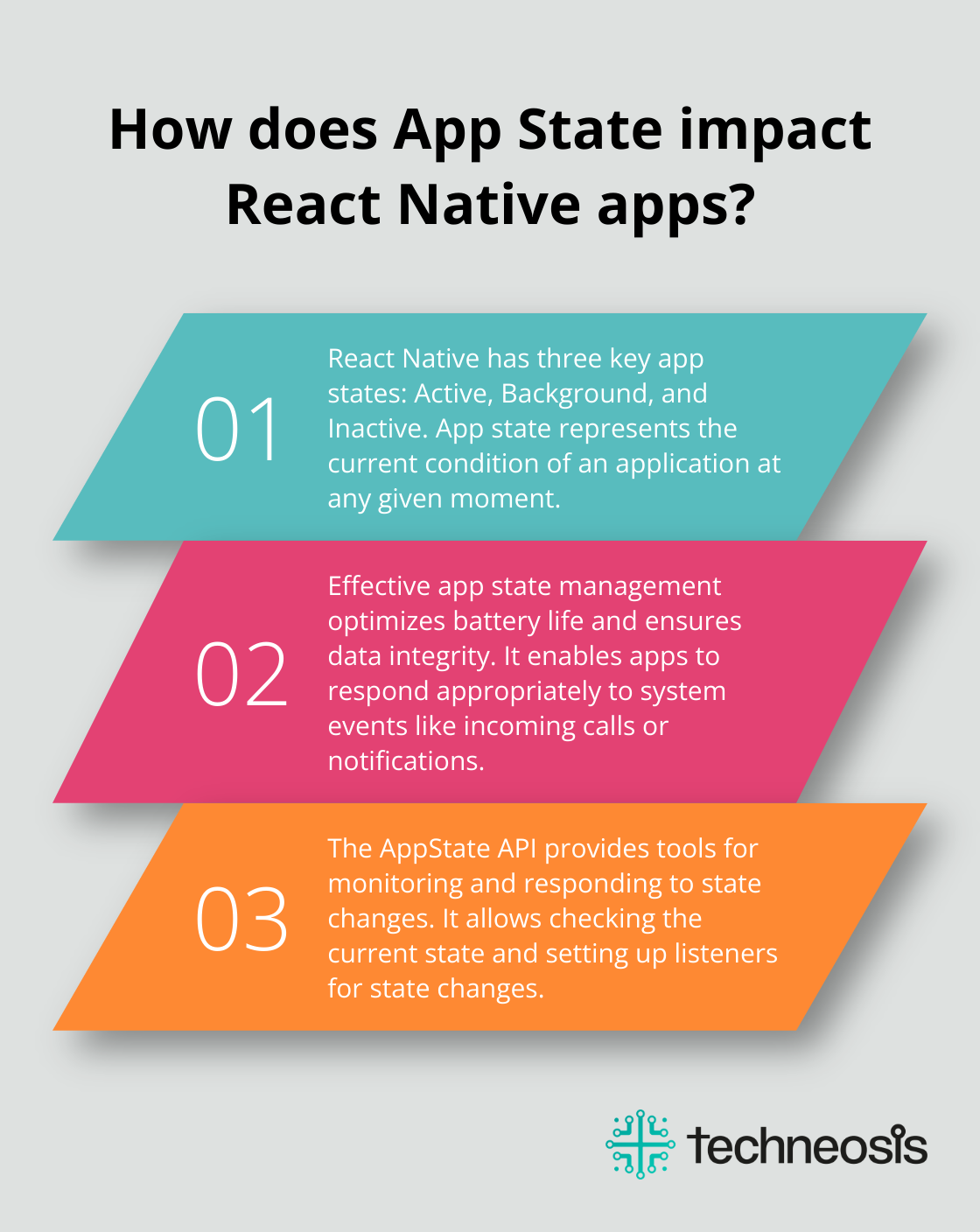
As we move forward, we’ll explore how to implement React Native app state change listeners and leverage them for practical use cases. Let’s start by looking at the specifics of implementing app state listeners in React Native.
Choosing the Right Framework
When developing a mobile app, it’s crucial to explore top mobile app dev frameworks to find the perfect fit for your project. Understanding the pros, cons, and current trends in mobile app development frameworks can help you make an informed decision and ensure your app state management strategy aligns with your chosen framework’s capabilities.
How to Implement App State Listeners in React Native
Understanding the AppState Module
React Native’s AppState module provides a powerful tool for monitoring and responding to app state changes. The AppState can tell you if the app is in the foreground or background, and notify you when the state changes. To start, import the AppState module:
javascript
import { AppState } from ‘react-native’;
This module allows you to track whether your app is active, in the background, or inactive.
Setting Up Listeners with useEffect
Implement app state listeners using React’s useEffect hook. This approach ensures proper setup and cleanup of listeners:
javascript
useEffect(() => {
const subscription = AppState.addEventListener(‘change’, handleAppStateChange);
return () => {
subscription.remove();
};
}, []);
The subscription object returned by addEventListener allows for easy removal of the listener when the component unmounts.
Handling Different App States
Define a function to handle app state changes. This function will execute whenever the app’s state transitions:
javascript
const handleAppStateChange = (nextAppState) => {
if (nextAppState === ‘active’) {
// App is in the foreground
refreshData();
} else if (nextAppState === ‘background’) {
// App is in the background
saveAppState();
} else if (nextAppState === ‘inactive’) {
// App is in a transitional state
prepareForBackground();
}
};
Optimizing for Performance
To maintain optimal performance, follow these best practices:
- Remove listeners when components unmount to prevent memory leaks.
- Use AppState.currentState to initialize your app’s state correctly (especially after a cold start).
- Avoid heavy operations during the ‘inactive’ state, which is typically brief.
Practical Applications of App State Management
App state management has numerous practical applications. Exploring tips, libraries, and examples can improve the performance, scalability, and efficiency of React Native state management. Some applications include:
- Pause video playback when the app moves to the background
- Trigger data synchronization when the app becomes active
- Manage push notifications effectively (regardless of the app’s state)
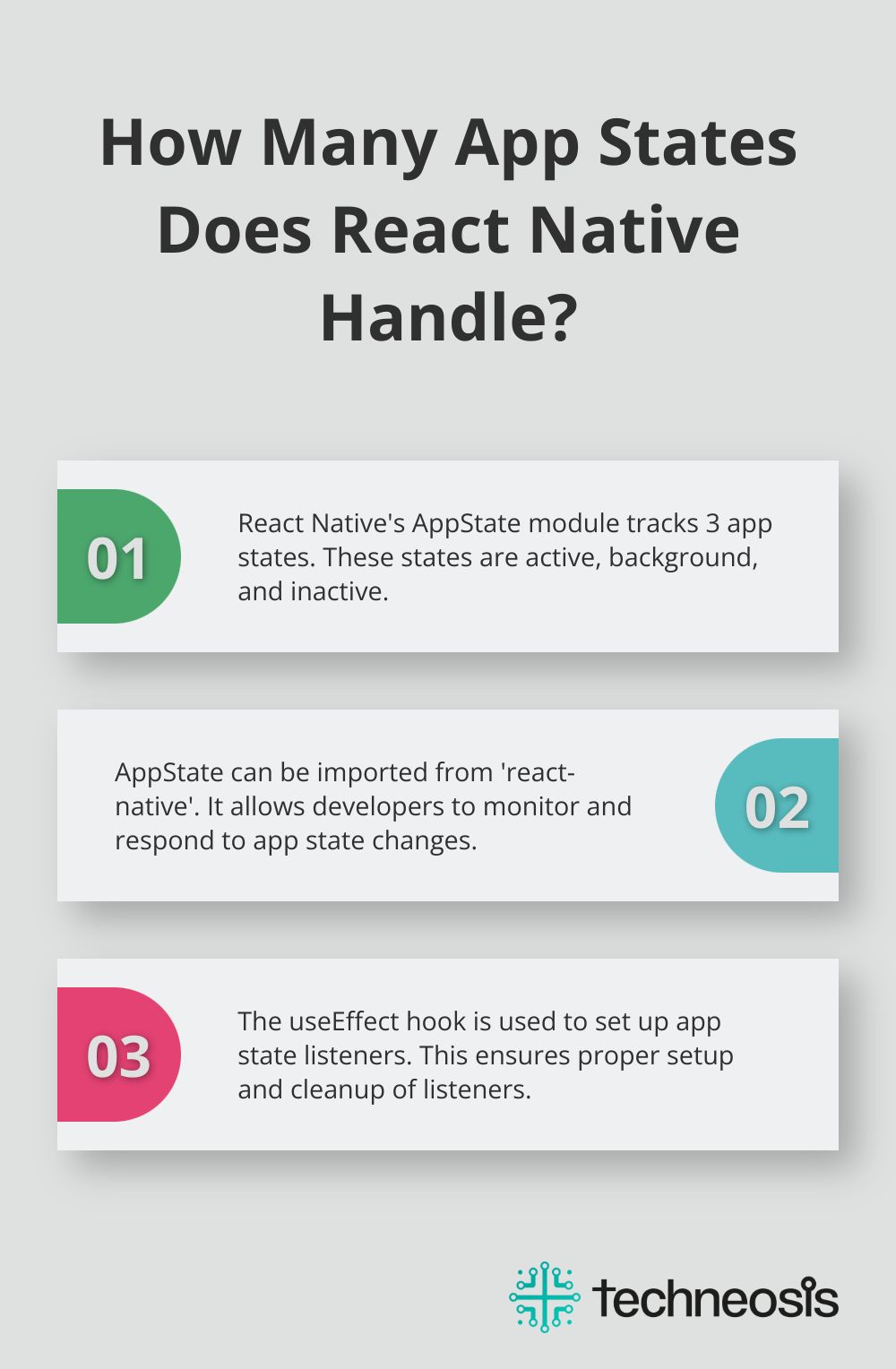
Implementing these features can significantly enhance user experience and app efficiency.
The next chapter will explore specific use cases for app state management, demonstrating how to apply these concepts in real-world scenarios.
Real-World App State Management Techniques
Optimizing Background Tasks
We implemented an effective solution for a music streaming app that needed to continue playback when users switched to other apps. Our approach adjusted audio settings based on the app’s state:
javascript
const handleAppStateChange = (nextAppState) => {
if (nextAppState === ‘background’) {
AudioManager.configureBackgroundMode();
} else if (nextAppState === ‘active’) {
AudioManager.configureForegroundMode();
}
};
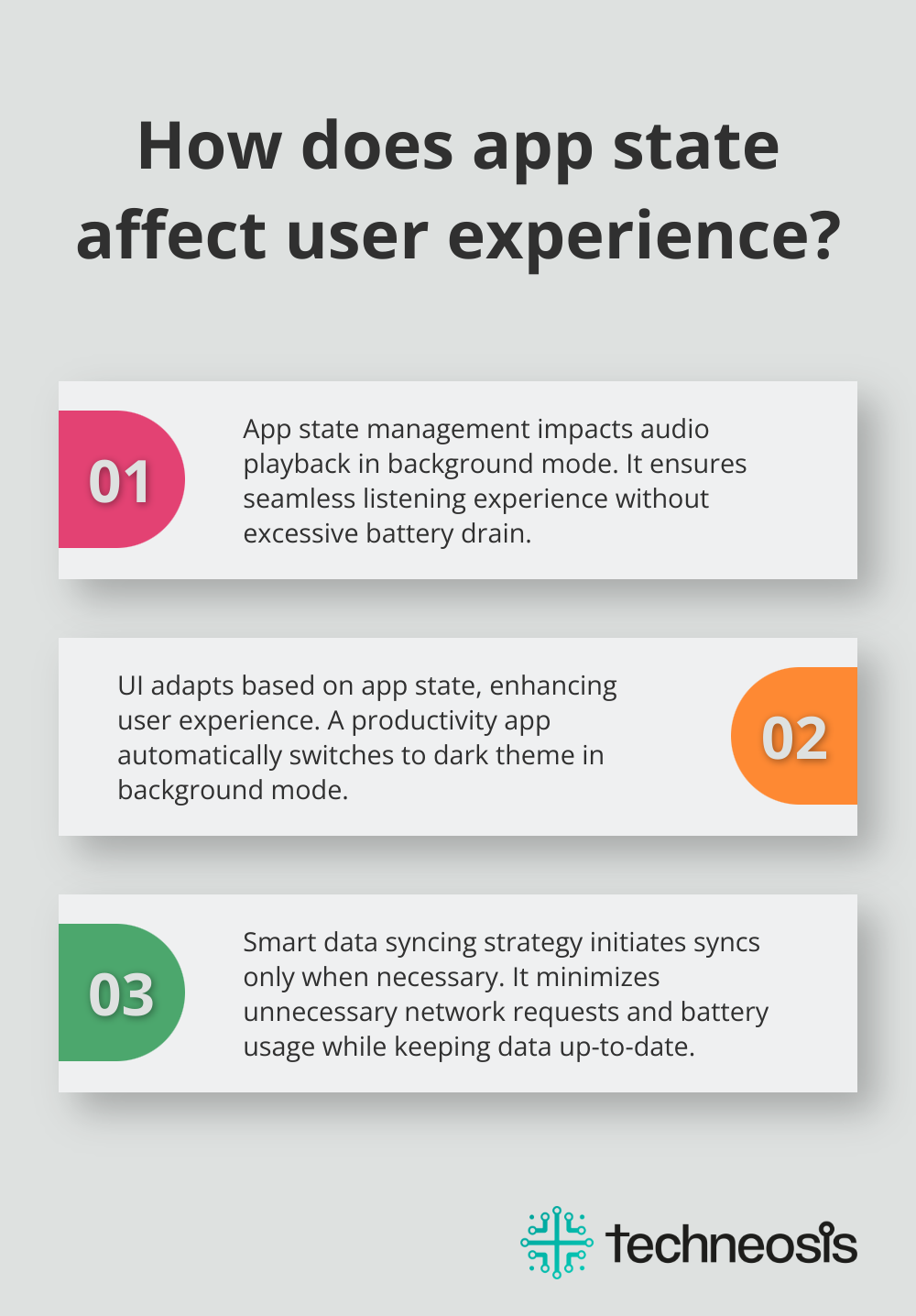
This method ensured a seamless listening experience without excessive battery drain.
Dynamic UI Updates
Adapting the UI based on app state can enhance user experience significantly. For a productivity app, we added a feature that automatically switched to a dark theme when the app entered background mode:
javascript
const updateUIForAppState = (nextAppState) => {
if (nextAppState === ‘active’) {
ThemeManager.setLightMode();
} else {
ThemeManager.setDarkMode();
}
};
This simple adjustment received positive user feedback, demonstrating the impact of small UI changes. Dynamic UI Updates based on app state can be implemented to change theme mode based on user’s local time in React Native.
Smart Data Syncing
Efficient data syncing is essential for apps that rely on up-to-date information. We created a smart syncing strategy for a task management app that initiated syncs only when necessary:
javascript
const handleAppStateChange = async (nextAppState) => {
if (nextAppState === ‘active’) {
const lastSyncTime = await AsyncStorage.getItem(‘lastSyncTime’);
const currentTime = Date.now();
if (currentTime – lastSyncTime > 300000) { // 5 minutes
syncData();
AsyncStorage.setItem(‘lastSyncTime’, currentTime.toString());
}
}
};
This approach minimized unnecessary network requests and battery usage while ensuring users always had the latest data when opening the app.
Performance Optimization
To maintain optimal performance, try these best practices:
- Remove listeners when components unmount to prevent memory leaks.
- Use AppState.currentState to initialize your app’s state correctly (especially after a cold start).
- Avoid heavy operations during the ‘inactive’ state, which is typically brief.
Practical Applications
App state management has numerous practical applications. Some examples include:
- Pausing video playback when the app moves to the background
- Triggering data synchronization when the app becomes active
- Managing push notifications effectively (regardless of the app’s state)
Implementing these features can significantly improve user experience and app efficiency.
Final Thoughts
This post explored the intricacies of handling React Native app state changes. We covered essential strategies for creating responsive and efficient mobile applications, from understanding app state basics to implementing practical solutions. Effective app state management forms the cornerstone of delivering a seamless user experience, allowing developers to create applications that respond intelligently to user actions and system events.
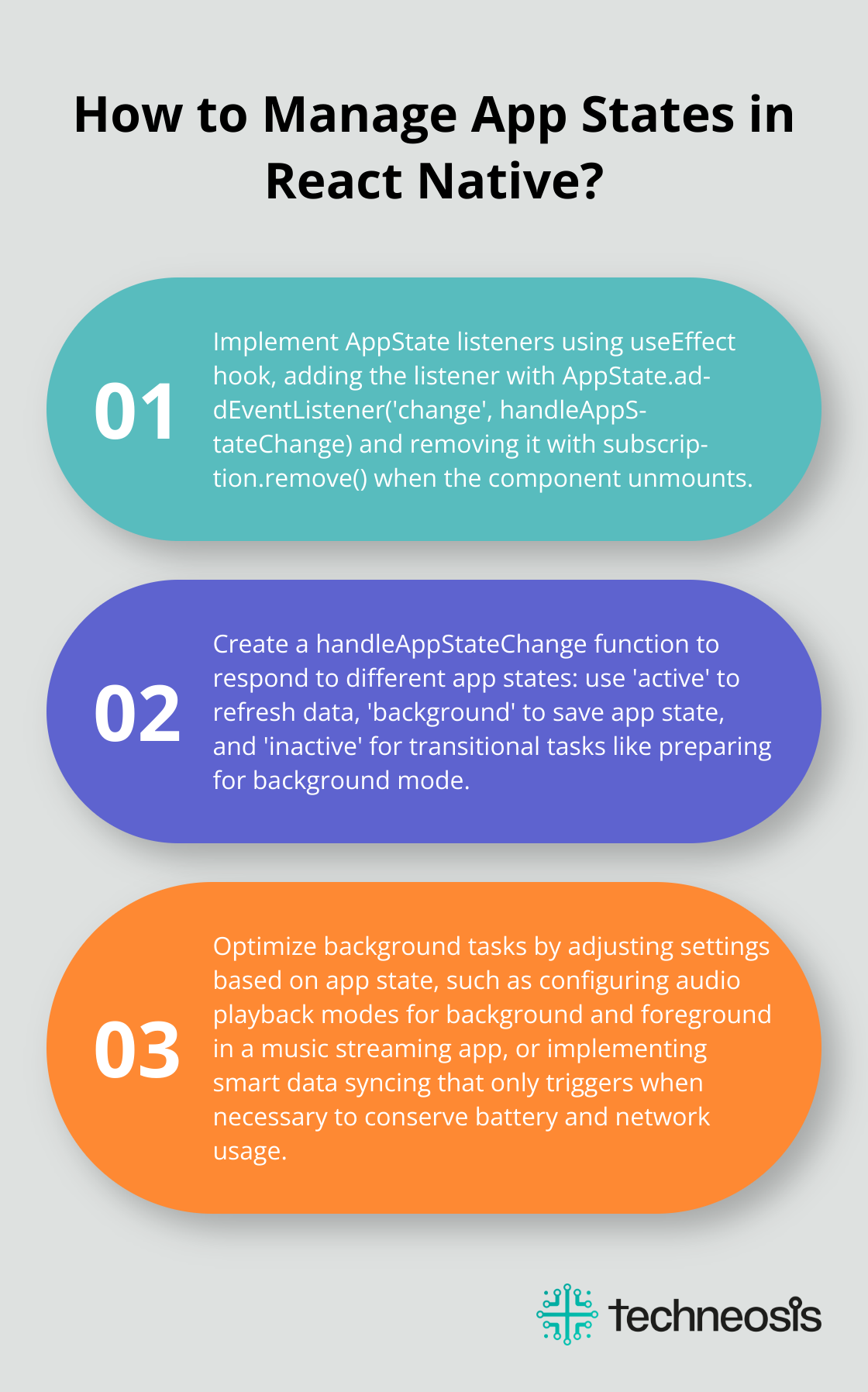
Developers can optimize app performance through state handling by removing listeners when components unmount, using AppState.currentState for correct initialization, and avoiding heavy operations during brief transitional states. Smart syncing strategies balance data freshness with battery life and network usage, creating apps that feel responsive and reliable in both foreground and background states. These techniques significantly enhance an app’s user experience and overall performance.
At Techneosis, we develop strategic technology solutions aligned with business goals. Our expertise in React Native development (including advanced app state management techniques) can help create mobile applications that stand out in today’s competitive market. We guide clients through every step of the process, whether optimizing existing apps or building new ones from scratch.