Back-end mobile app development is the backbone of any successful mobile application. It’s the hidden powerhouse that drives functionality, manages data, and ensures seamless user experiences.
At Techneosis, we’ve seen firsthand how proper back-end practices can make or break an app’s performance and scalability. In this post, we’ll explore the best practices that can elevate your mobile app’s back-end infrastructure to new heights.
Selecting the Ideal Tech Stack
Choosing the right technology stack determines the success of your mobile app. The best choice depends on your project requirements, scalability needs, and team expertise. Let’s explore the key factors to consider when selecting your tech stack.
Programming Languages and Frameworks
Python, Java, and Node.js stand out as popular choices for mobile app back-ends. Each language offers unique advantages:
- Python: Known for simplicity and extensive libraries (ideal for rapid development)
- Java: Offers robust performance (widely used in enterprise applications)
- Node.js: Excels in handling concurrent connections (suitable for real-time applications)
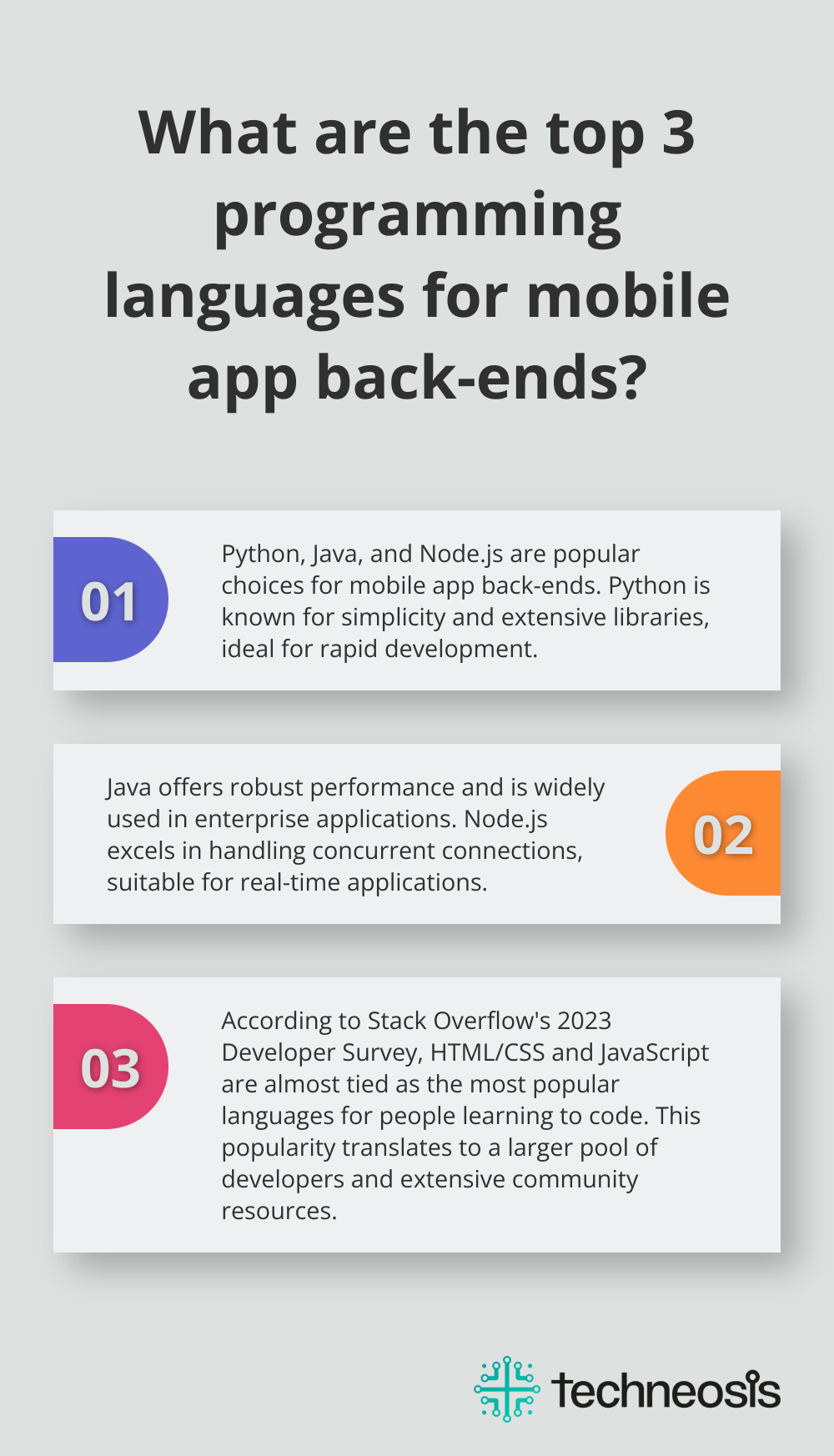
For frameworks, consider these widely adopted options:
- Django (Python): Provides a full-featured environment with built-in admin interfaces
- Spring Boot (Java): Offers excellent support for microservices
- Express.js (Node.js): Lightweight and flexible, perfect for building RESTful APIs
Scalability and Performance Considerations
Scalability plays a critical role in tech stack selection. For rapid growth expectations, focus on technologies that scale horizontally with ease. Node.js and Go excel at building highly concurrent systems.
For database solutions, NoSQL options like MongoDB or Cassandra handle large volumes of unstructured data more efficiently than traditional relational databases.
Performance-wise, compiled languages (Go or Rust) offer advantages for compute-intensive tasks. However, interpreted languages (Python or Ruby) might suit projects where development speed takes priority.
Developer Expertise and Community Support
The availability of skilled developers and strong community support impacts your project’s success. According to Stack Overflow’s 2023 Developer Survey, HTML/CSS and JavaScript are almost tied as the most popular languages for people learning to code. This popularity translates to a larger pool of developers and extensive community resources.
Consider your team’s learning curve. If your developers excel in a particular language, it might prove more efficient to stick with it rather than adopt a new technology stack (even if theoretically superior).
Community support proves essential for troubleshooting and staying updated with best practices. Look for technologies with active GitHub repositories, comprehensive documentation, and regular updates. Node.js, for example, boasts a vibrant ecosystem with over 1.3 million packages on npm, providing solutions for almost any functionality you might need.
The most successful projects often leverage a combination of established technologies and cutting-edge tools. We recommend starting with a well-established core stack and gradually incorporating newer technologies as your team gains expertise and your app’s needs evolve.
As we move forward, let’s examine how to design a robust API architecture that will serve as the foundation for your mobile app’s back-end infrastructure.
How to Build a Robust API Architecture
RESTful vs GraphQL: Choosing Your API Paradigm
A well-designed API forms the foundation of any successful mobile app. It creates a seamless, efficient, and secure communication channel between your app and its backend.
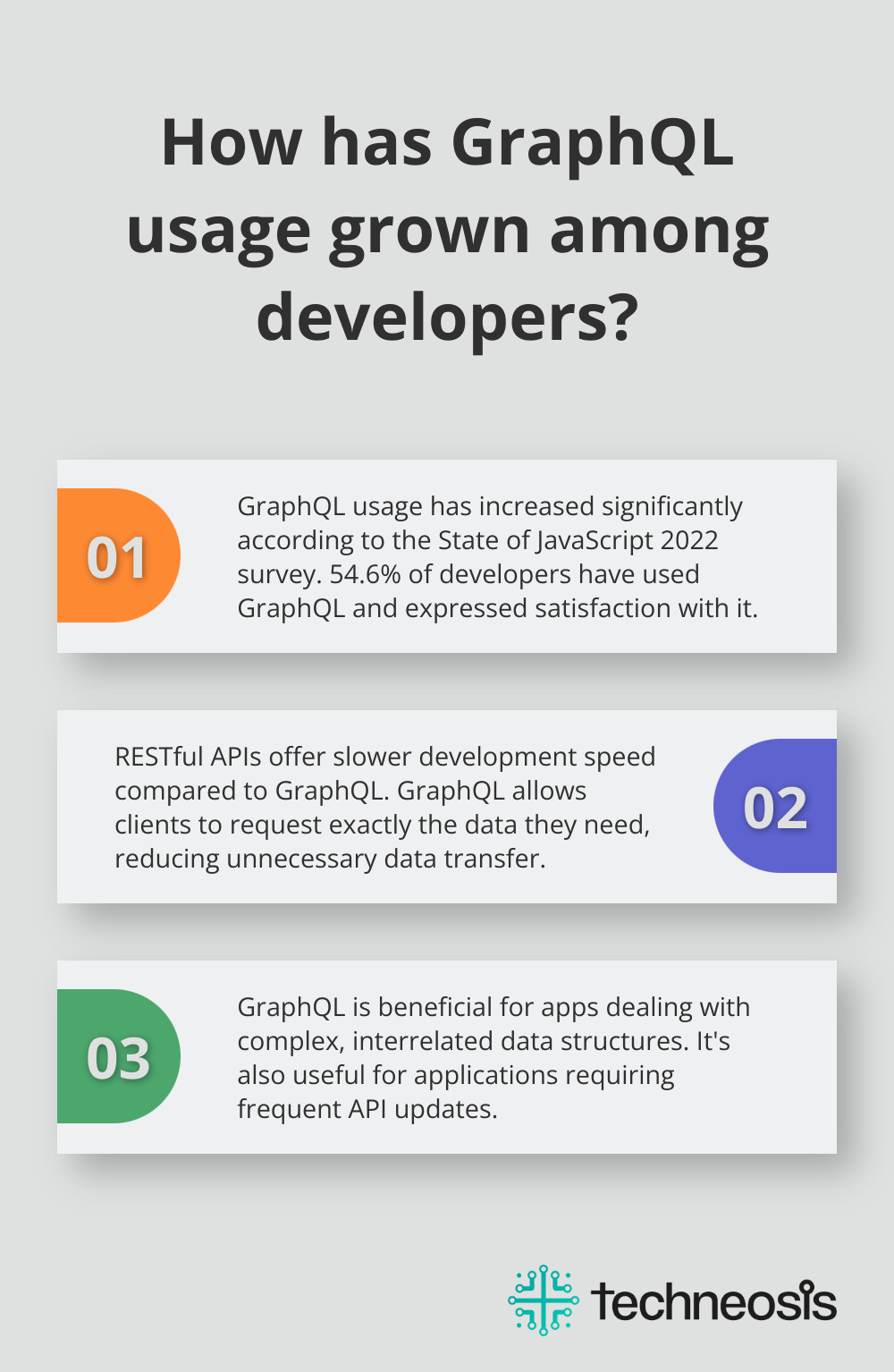
When designing APIs, developers often debate between RESTful APIs and GraphQL. Each option has its strengths, and your choice should align with your app’s specific needs.
RESTful APIs offer a slower development speed compared to GraphQL. They excel in straightforward CRUD operations and provide clear endpoints for each resource. However, they can sometimes lead to over-fetching or under-fetching of data, which may impact performance.
GraphQL provides more flexibility. It allows clients to request exactly the data they need, reducing unnecessary data transfer. This can benefit mobile apps where bandwidth is a concern. The State of JavaScript 2022 survey revealed that GraphQL usage has grown significantly, with 54.6% of developers having used it and expressing satisfaction.
For apps dealing with complex, interrelated data structures or requiring frequent API updates, GraphQL might be the better choice. For simpler applications or when working with legacy systems, RESTful APIs remain a solid option.
Securing Your API: Authentication and Authorization
API security is non-negotiable. Implementing robust authentication and authorization mechanisms protects your users’ data and your app’s integrity.
JSON Web Tokens (JWTs) have gained popularity for authentication due to their stateless nature and ability to securely transmit information between parties. They suit mobile apps well as they can be easily stored and transmitted.
Implement authorization using role-based access control (RBAC) or attribute-based access control (ABAC). These methods ensure users can only access permitted resources based on their role or specific attributes.
Rate limiting is also essential to prevent API abuse. Implement it at the API gateway level to protect your backend from potential DDoS attacks or excessive use by a single client.
Optimizing Performance: Efficient Data Transfer
API performance can significantly impact your app’s user experience. Here are strategies to optimize data transfer and minimize network requests:
Use HTTP caching headers to allow clients to store responses locally. This can reduce the number of requests made to your server.
Apply GZIP compression to reduce the size of your API responses.
Implement pagination for large datasets to limit the amount of data transferred in a single request.
Allow clients to make multiple API calls in a single HTTP request through batch requests. This can help in unreliable network conditions.
Consider alternatives to JSON, like Protocol Buffers, for even more efficient data serialization.
Future-Proofing Your API
As your app evolves, your API needs to adapt. Design your API with versioning in mind from the start. This allows you to introduce new features or make changes without breaking existing client integrations.
Document your API thoroughly. Clear, comprehensive documentation makes it easier for developers to integrate with your API and reduces support overhead. Tools like Swagger can automate much of this process.
Monitor your API’s performance and usage patterns. This data can inform future optimizations and help you identify potential issues before they impact users.
The next step in building a robust backend for your mobile app involves effective data management and storage strategies. Let’s explore how to select the right database solutions and implement efficient data models in the next section.
Mastering Data Management for Mobile Apps
Effective data management forms the backbone of high-performing mobile apps. By understanding data types, choosing appropriate storage solutions, implementing security measures, and ensuring efficient synchronization, you can significantly impact app performance and user satisfaction. This chapter explores strategies to optimize your app’s data infrastructure.
Selecting the Optimal Database
The choice of database solution can make or break your app’s success. Consider these options for most mobile apps:
SQLite: Perfect for local storage on devices. Its lightweight nature and zero configuration make it ideal for offline-first apps. SQLite takes care of file-level locking, allowing many threads to read while one can write.
PostgreSQL: A powerful relational database that handles complex queries and maintains data integrity. It excels for apps with intricate data relationships.
MongoDB: A popular NoSQL option offering flexibility in data modeling and horizontal scalability. It suits apps with rapidly changing data structures.
Redis: An in-memory data structure store for caching and real-time data processing. It often complements a primary database to boost performance.
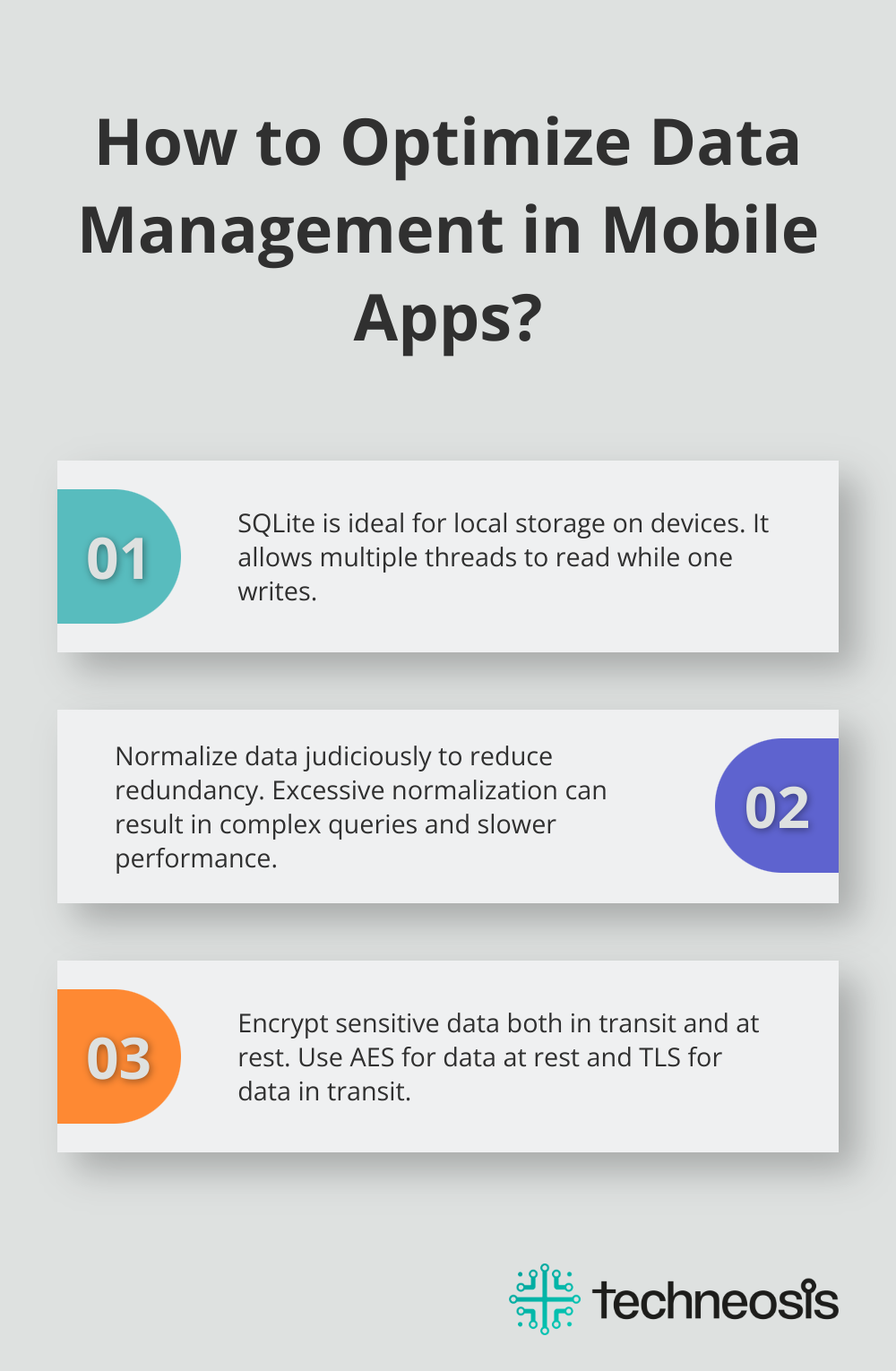
Your app’s data structure, expected growth, and query patterns should guide your choice. Apps dealing with highly structured data and complex relationships might benefit from a relational database like PostgreSQL. For large volumes of unstructured data, a NoSQL solution like MongoDB could prove more appropriate.
Implementing Efficient Data Models
Proper data modeling plays a key role in app performance. These strategies prove effective:
Normalize data judiciously. While normalization reduces redundancy, excessive normalization can result in complex queries and slower performance.
Use indexing strategically. Indexes can speed up read operations significantly (at the cost of slower write operations and increased storage requirements).
Implement caching mechanisms. Tools like Redis can dramatically reduce database load by caching frequently accessed data.
Consider denormalization for read-heavy operations. In some cases, data duplication leads to faster queries and improved user experience.
Choose appropriate data types. The right data type for each field optimizes storage and improves query performance.
Your data model should evolve based on your app’s specific needs and usage patterns.
Prioritizing Data Security and Compliance
Data security stands as a non-negotiable aspect of mobile app development. Implement these key practices:
Encrypt sensitive data both in transit and at rest. Use industry-standard encryption algorithms (AES for data at rest, TLS for data in transit).
Implement proper access controls. Role-based access control (RBAC) ensures users can only access authorized data. The first step for implementing RBAC for an application is to define the app roles and assign users or groups to them.
Perform regular data backups. Implement automated backup solutions and test restore processes frequently.
Maintain compliance with relevant regulations. Depending on your app’s nature and user base, you may need to adhere to GDPR, CCPA, or HIPAA.
Conduct regular security audits. Proactively identify and address potential vulnerabilities in your data management systems.
Implement secure API practices. Use OAuth 2.0 for authentication and implement rate limiting to prevent abuse.
Practice transparency about data usage. Clearly communicate to users how you collect, use, and protect their data.
Data management directly impacts user experience, app performance, and business success. Careful selection of database solutions, optimization of data models, and prioritization of security build a robust foundation for your mobile app.
Final Thoughts
Back-end mobile app development forms the foundation of successful applications. Developers create robust, scalable, and secure systems that drive exceptional user experiences by following best practices. The process doesn’t end with initial development; continuous monitoring and optimization maintain peak performance. Regular analysis of API usage patterns, database query performance, and overall system health allows for proactive improvements.
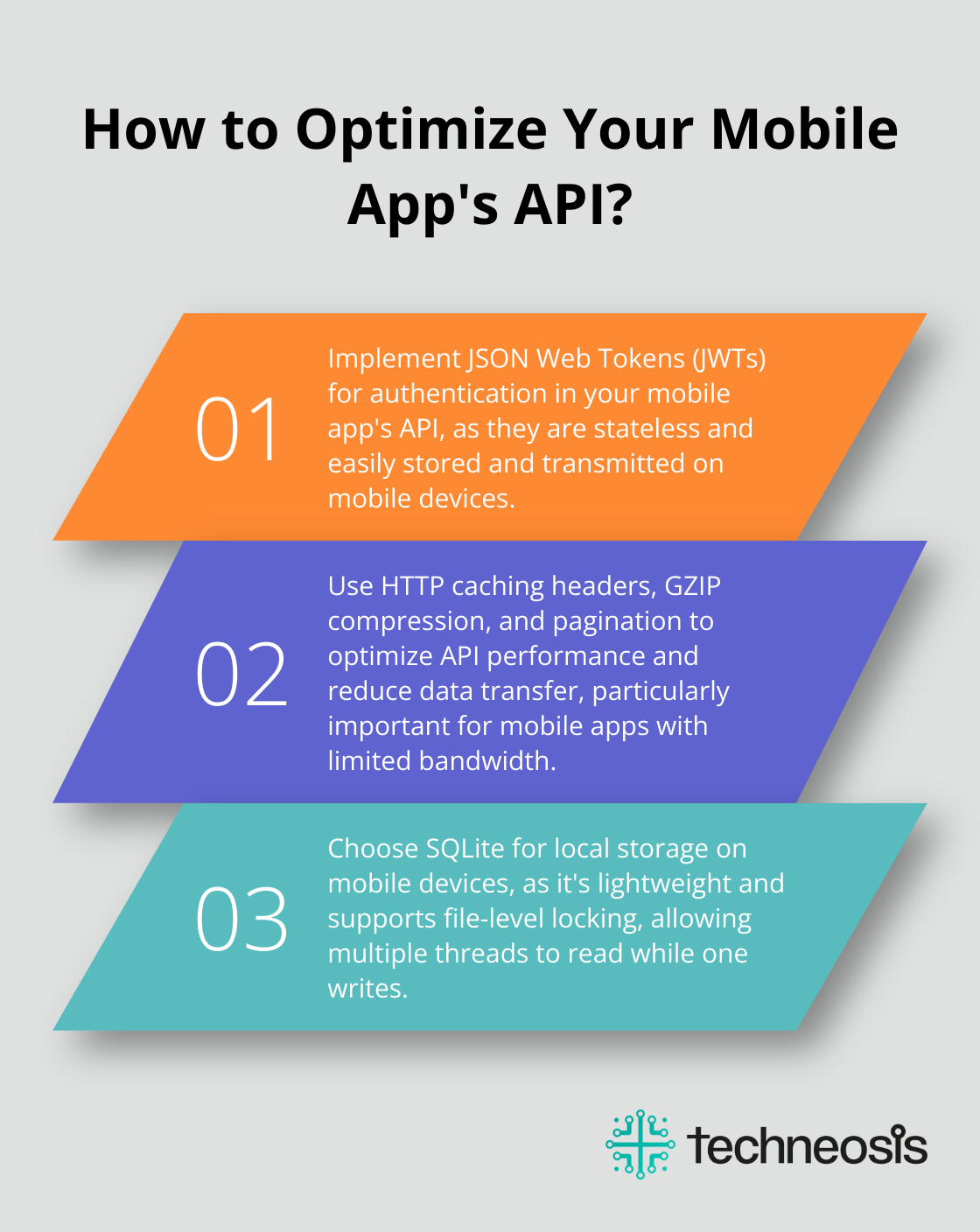
The mobile app landscape evolves rapidly, and adaptability is essential. Developers must stay current with emerging technologies, security protocols, and user expectations. This flexibility ensures that apps remain competitive and continue to meet user demands. Refactoring code, updating APIs, and migrating to new database solutions may become necessary as needs change.
We at Techneosis understand the complexities of back-end development for mobile apps. Our team can guide you through the process, from initial planning to ongoing optimization. We tailor solutions to specific business needs, ensuring that your mobile app’s back-end infrastructure aligns with your long-term goals and supports your growth.